用Python实现迷宫游戏
- 获取方式看文末-
实现迷宫游戏的一般思路如下:
1. 创建一个二维数组,代表迷宫的地图。其中0代表可通行的路径,1代表障碍物。
2. 随机生成起点和终点的位置。
3. 通过算法(如深度优先搜索)随机生成迷宫地图,确保起点和终点可以互相到达。
4. 在游戏界面上绘制迷宫地图,同时在起点和终点的位置上添加标识。
5. 监听用户键盘输入,根据输入移动角色。如果角色到达终点,游戏结束。
下面是一个Python实现迷宫游戏的示例代码:
import pygame
import random
定义迷宫地图的宽和高
MAP_WIDTH = 20
MAP_HEIGHT = 20```
**定义地图上的元素**
```py
EMPTY = 0
WALL = 1
START = 2
END = 3```
**定义角色的移动方向**
```py
UP = 0
DOWN = 1
LEFT = 2
RIGHT = 3
初始化pygame
``py
pygame.init()```
创建游戏窗口
screen = pygame.display.set_mode((MAP_WIDTH * 20, MAP_HEIGHT * 20))
pygame.display.set_caption("迷宫游戏")
定义迷宫地图
map_data = [[EMPTY for y in range(MAP_HEIGHT)] for x in range(MAP_WIDTH)]
随机生成起点和终点的位置
start_pos = (random.randint(0, MAP_WIDTH - 1), random.randint(0, MAP_HEIGHT - 1))
end_pos = (random.randint(0, MAP_WIDTH - 1), random.randint(0, MAP_HEIGHT - 1))```
**确保起点和终点不在同一个位置**
```py
while start_pos == end_pos:
end_pos = (random.randint(0, MAP_WIDTH - 1), random.randint(0, MAP_HEIGHT - 1))
设置起点和终点的位置
map_data[start_pos[0]][start_pos[1]] = START
map_data[end_pos[0]][end_pos[1]] = END
生成迷宫地图
def generate_map(x, y):
# 如果当前位置已经访问过,则返回
if map_data[x][y] != EMPTY:
return```
**设置当前位置为障碍物**
```py
map_data[x][y] = WALL
随机生成下一个位置的方向
directions = [(0, -1), (0, 1), (-1, 0), (1, 0)]
random.shuffle(directions)
递归生成迷宫
for direction in directions:
next_x = x + direction[0] * 2
next_y = y + direction[1] * 2
if next_x 60 0 or next_x 62= MAP_WIDTH or next_y 60 0 or next_y 62= MAP_HEIGHT:
continue
generate_map(next_x, next_y)
生成迷宫地图
generate_map(random.randint(0, MAP_WIDTH - 1), random.randint(0, MAP_HEIGHT - 1))
定义角色的位置和方向
player_pos = start_pos
player_direction = RIGHT
游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
player_direction = UP
elif event.key == pygame.K_DOWN:
player_direction = DOWN
elif event.key == pygame.K_LEFT:
player_direction = LEFT
elif event.key == pygame.K_RIGHT:
player_direction = RIGHT
根据角色的方向移动角色
if player_direction == UP:
next_pos = (player_pos[0], player_pos[1] - 1)
elif player_direction == DOWN:
next_pos = (player_pos[0], player_pos[1] + 1)
elif player_direction == LEFT:
next_pos = (player_pos[0] - 1, player_pos[1])
elif player_direction == RIGHT:
next_pos = (player_pos[0] + 1, player_pos[1])
如果角色到达终点,游戏结束
if next_pos == end_pos:
print("游戏结束!")
pygame.quit()
exit()
如果下一个位置不是障碍物,则移动角色
if map_data[next_pos[0]][next_pos[1]] != WALL:
player_pos = next_pos
绘制游戏界面
screen.fill((255, 255, 255))
for x in range(MAP_WIDTH):
for y in range(MAP_HEIGHT):
if map_data[x][y] == WALL:
pygame.draw.rect(screen, (0, 0, 0), (x * 20, y * 20, 20, 20))
elif map_data[x][y] == START:
pygame.draw.rect(screen, (0, 255, 0), (x * 20, y * 20, 20, 20))
elif map_data[x][y] == END:
pygame.draw.rect(screen, (255, 0, 0), (x * 20, y * 20, 20, 20))
pygame.draw.rect(screen, (0, 0, 255), (player_pos[0] * 20, player_pos[1] * 20, 20, 20))
pygame.display.update()
pygame.time.delay(100)
运行以上代码,即可看到一个简单的迷宫游戏界面。按上下左右键可以移动角色,到达终点即可结束游戏。
- END -除上述资料外,还附赠全套Python学习资料,包含面试题、简历资料等具体看下方。
?福利? 全网最全《Python学习资料》免费赠送?!
学好 Python 不论是就业还是做副业赚钱都不错,但要学会 Python 还是要有一个学习规划。最后大家分享一份全套的 Python 学习资料,给那些想学习 Python 的小伙伴们一点帮助!
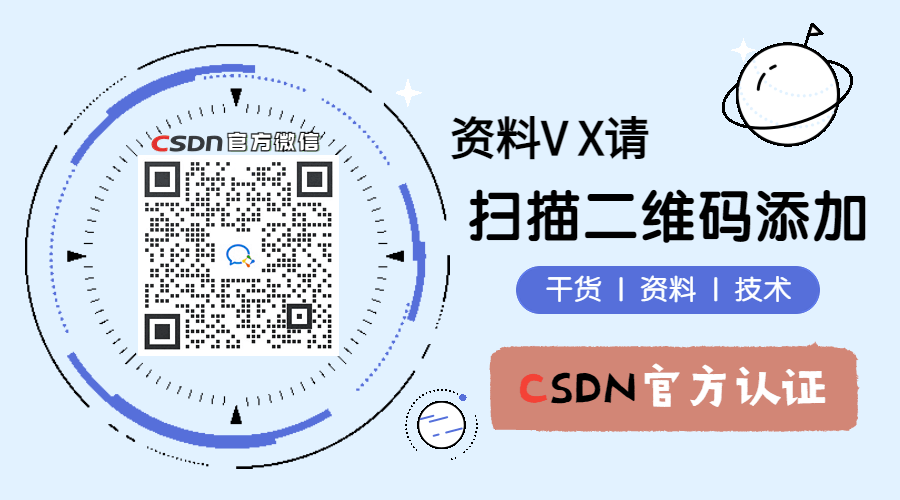
一、Python学习路线
二、Python基础学习
1. 开发工具
2. 学习笔记
3. 学习视频
三、Python小白必备手册
四、数据分析全套资源
五、Python面试集锦
1. 面试资料
2. 简历模板
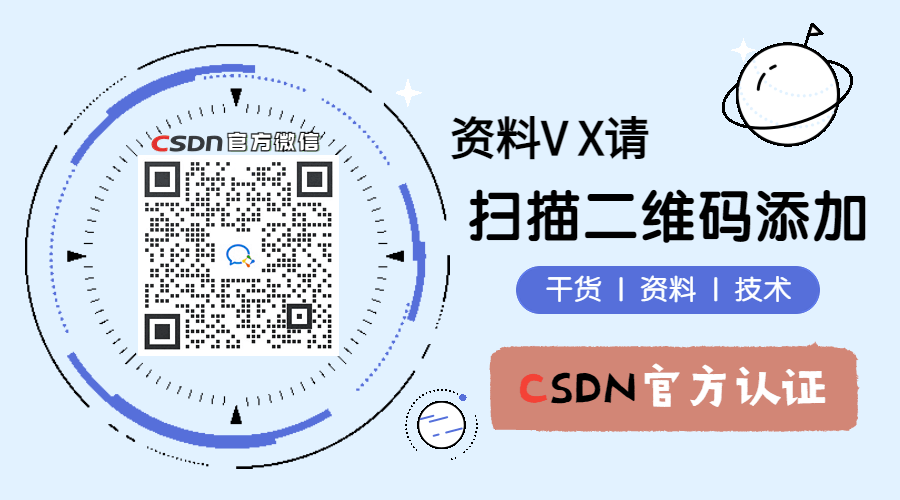
因篇幅有限,仅展示部分资料,添加上方即可获取